Short explanation
Moviee is a movie browsing platform that features various movie categories, a search function, and the ability to save favorite movies. Detailed movie pages provide comprehensive information about each film, including the plot, cast, reviews, release date, and the option to watch the trailer!
Goals
The goal of this project is primarily self-learning. After completing a bootcamp on Android development, I learned a lot, but what interested me the most was how to architect a codebase using MVVM and clean architecture. However, at that time, I didn’t fully understand the benefits of implementing these patterns in real-world projects. So, I decided to create one—the Moviee app.
Overall, I wanted to understand the practical benefits of these design patterns without any external pressure, deadlines, or constraints. This allows for continuous development and helps me see how these approaches help me as a developer.
Tech Stack
I’m using Kotlin for Android development, with the help of various dependencies to enable the app’s functionality. You can see the full dependencies used in this app from my Github Repository.
The architecture of this app complies with the following three principles:
-
By utilizing the ViewModel, this architecture achieves a more modular, testable, and maintainable codebase. It improves separation of concerns and establishes a clear distinction between UI logic and business logic/data operations.
-
Modularization enables the development of features in isolation, independent of other features. This approach provides benefits such as reusability, scalability, maintainability, reduced app size, and easier modification or replacement of specific features.
-
Clean Architecture strictly emphasizes a clear separation of concerns through distinct architectural layers: Presentation/UI Layer, Domain Layer, and Data Layer. This separation facilitates writing tests without dependencies on external frameworks or UI components, significantly enhancing testability.
Image above show the dependencies between module
The :app
module directly depends on :core
, :common-ui
, and :feature
, meaning it cannot function if any of them are missing. It also indirectly depends on :utility
if required.
The :feature
module directly depends on :core
and :common-ui
and indirectly depends on :utility
.
The :core
module indirectly depends on :utility
, whereas :utility
and :common-ui
have no dependencies.
Features
This app offers several features.
-
Explore Movies
: Browse through various categories and genres to discover movies that interest you. -
Detailed Information
: Access comprehensive details about each movie, including cast, release information, budget, and recommendations. -
Search Functionality
: Easily find specific movies using the search feature. -
Favorites List
: Save movies to your favorites for quick access later. -
Watch Trailers
: View trailers to get a glimpse of the movies before watching.
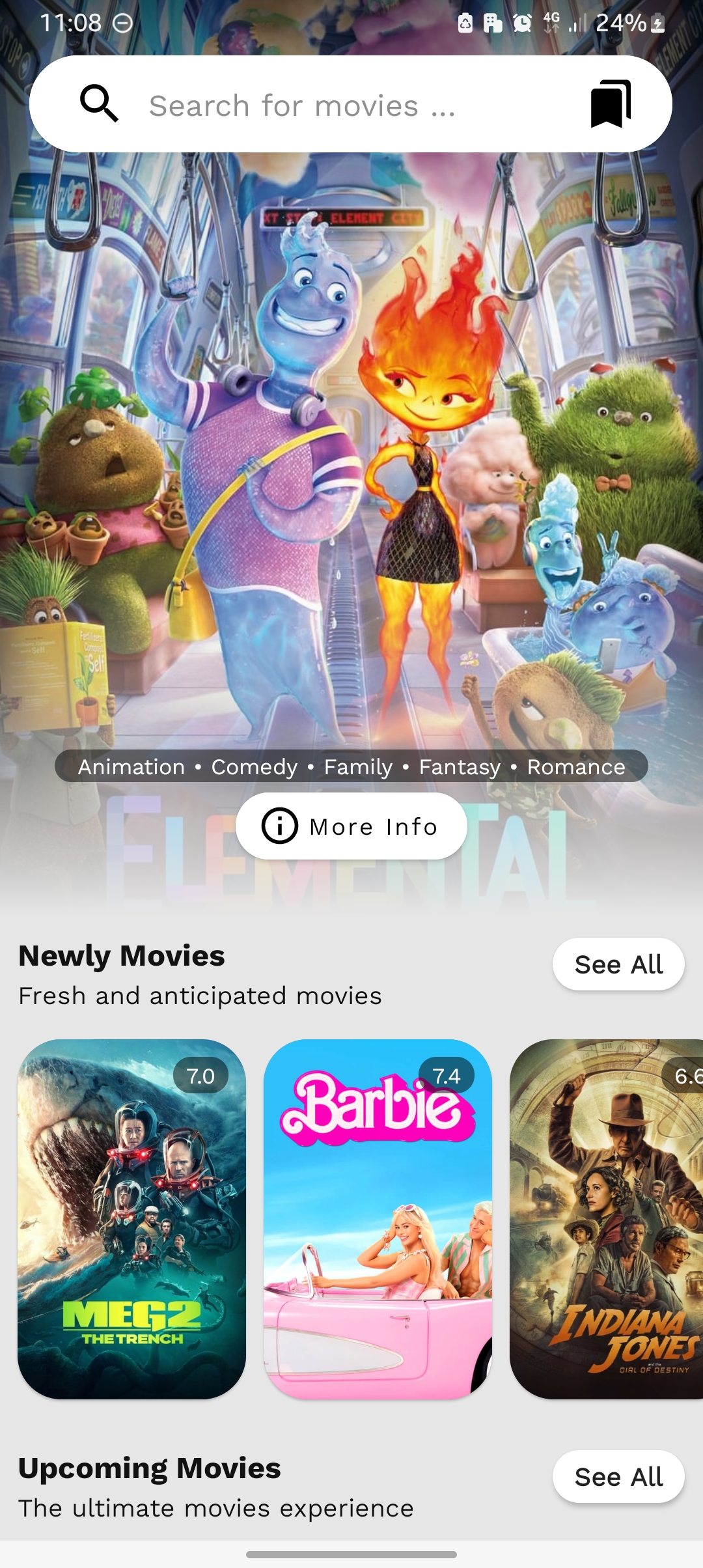
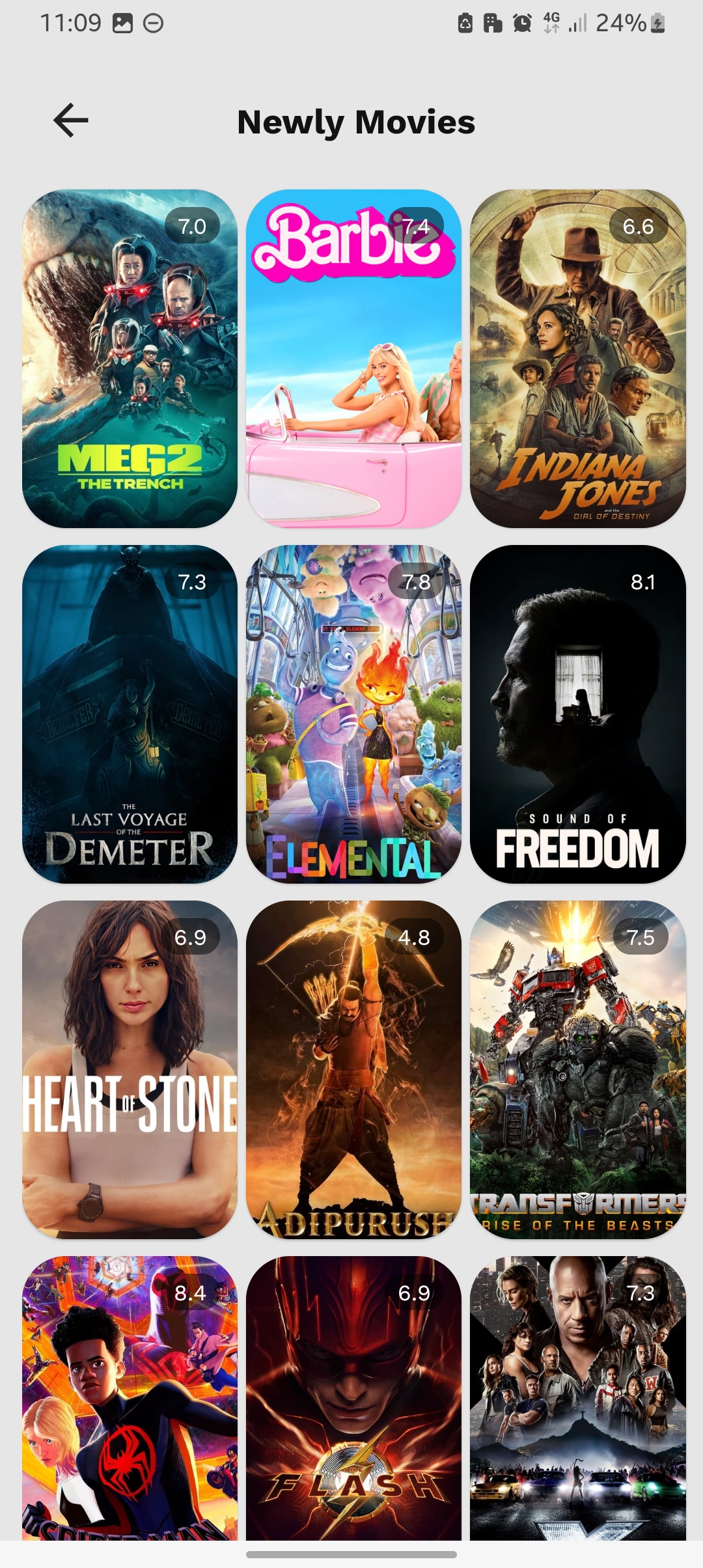
Showcases various categories such as Top Rated and Trending movies.
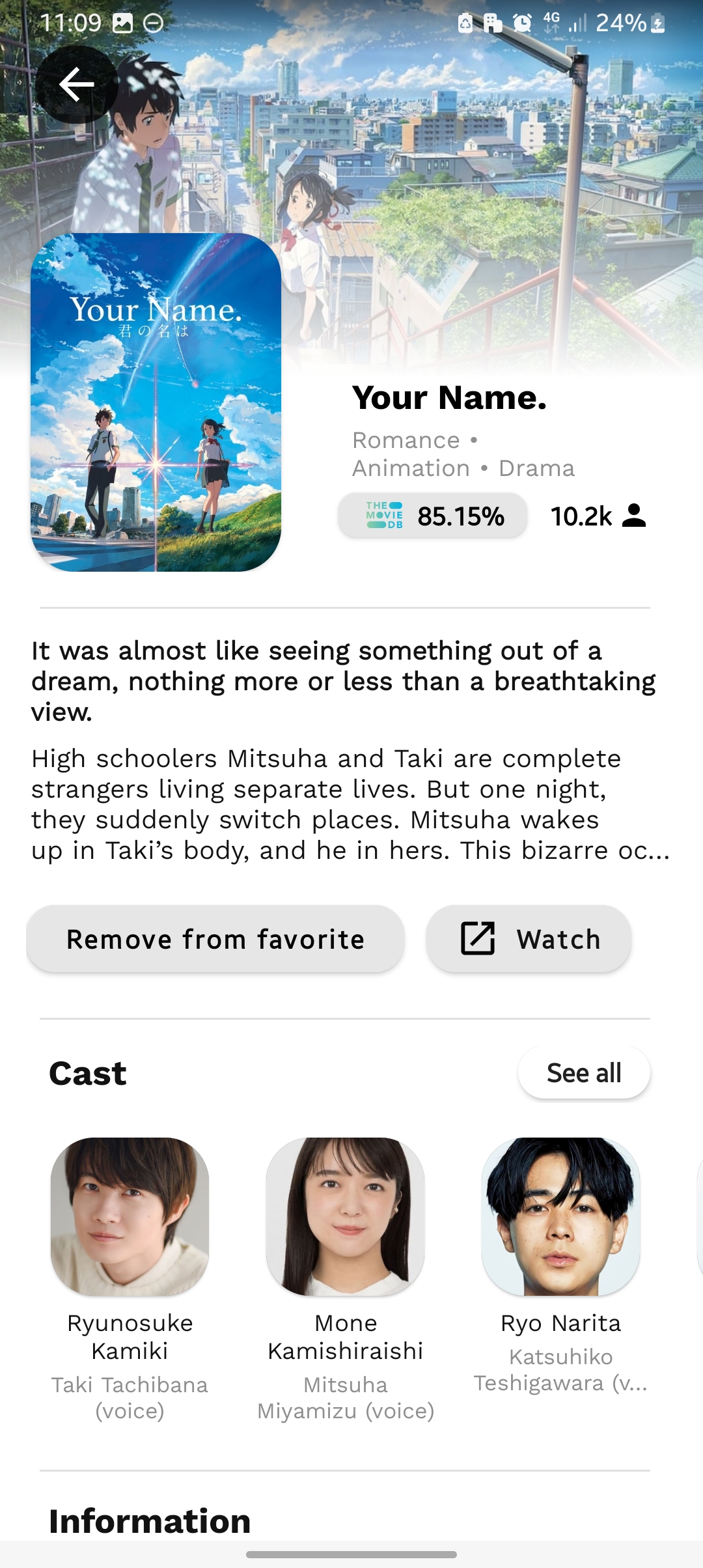
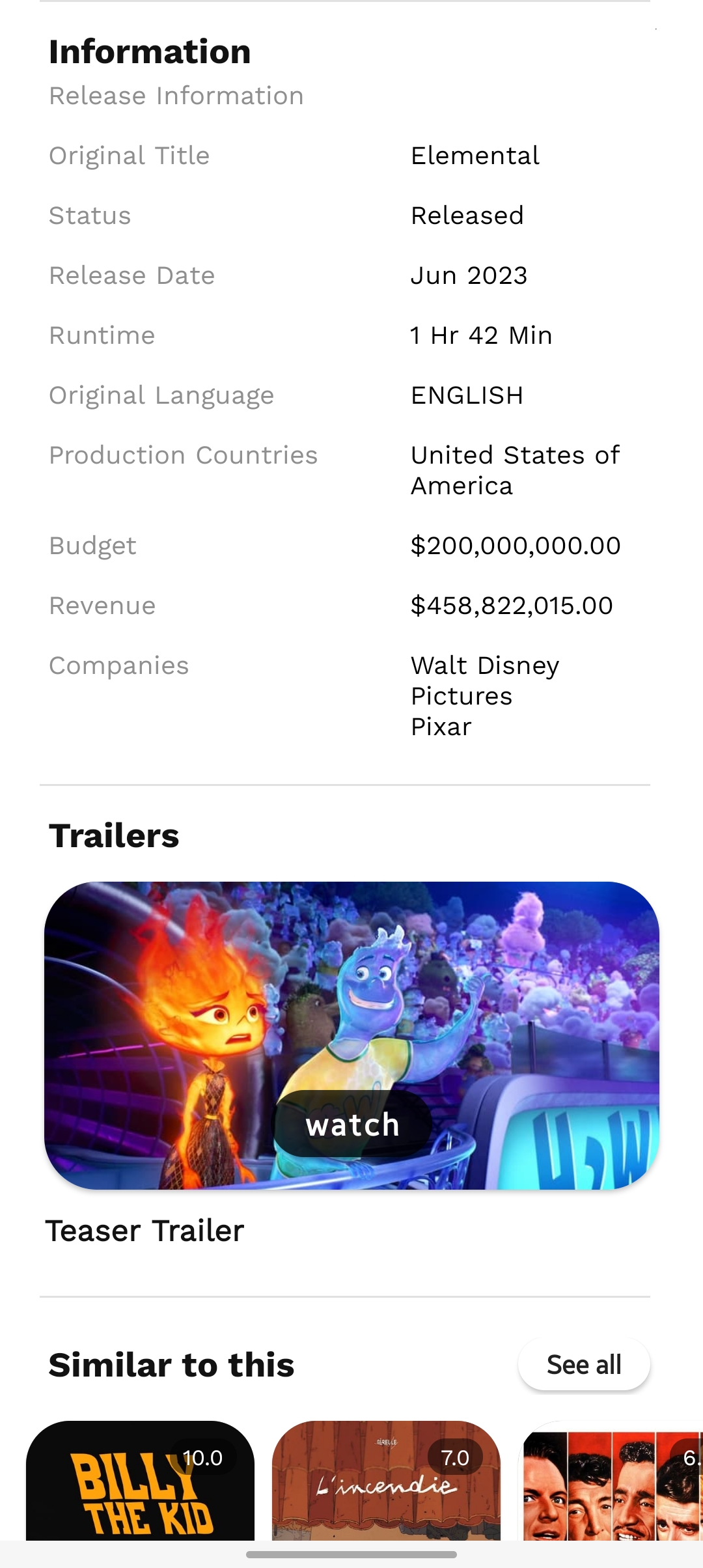
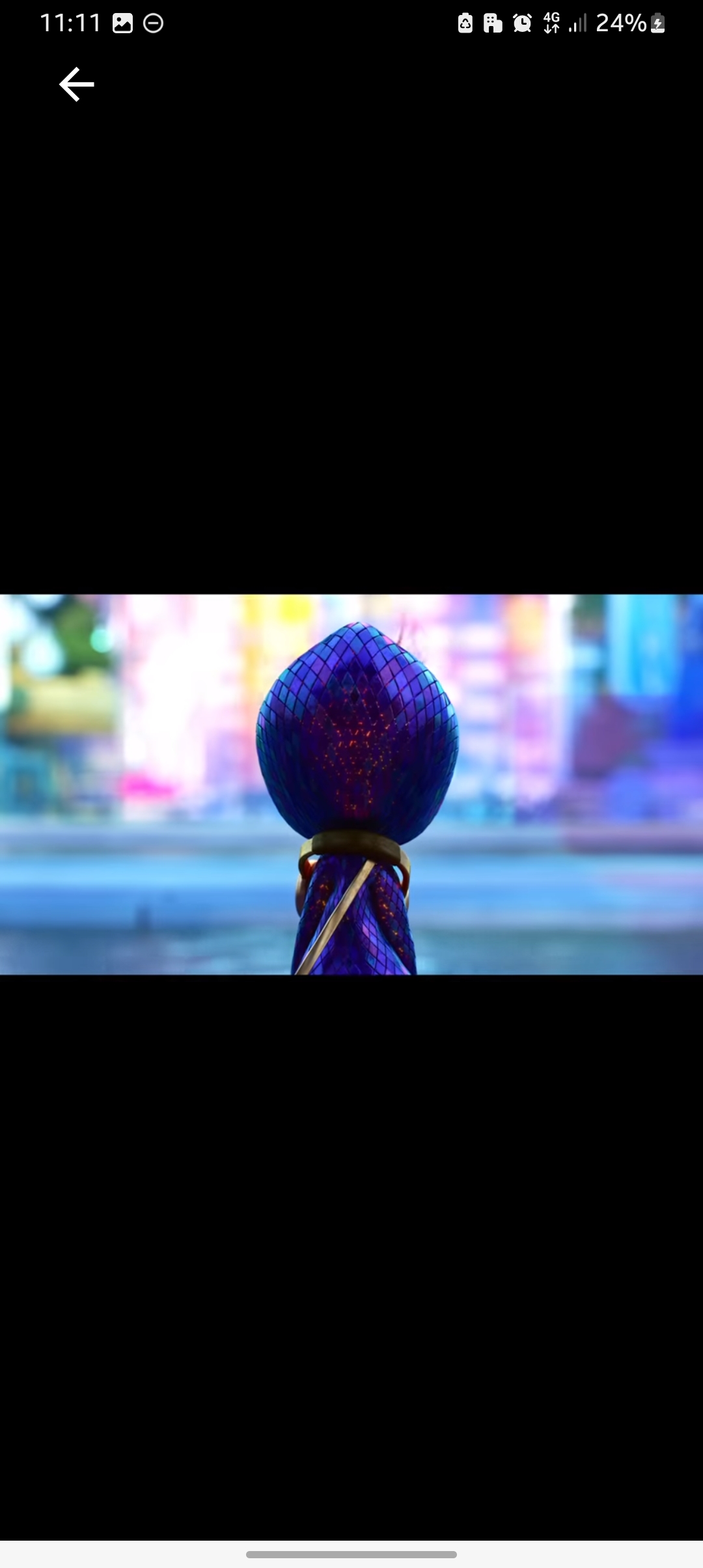
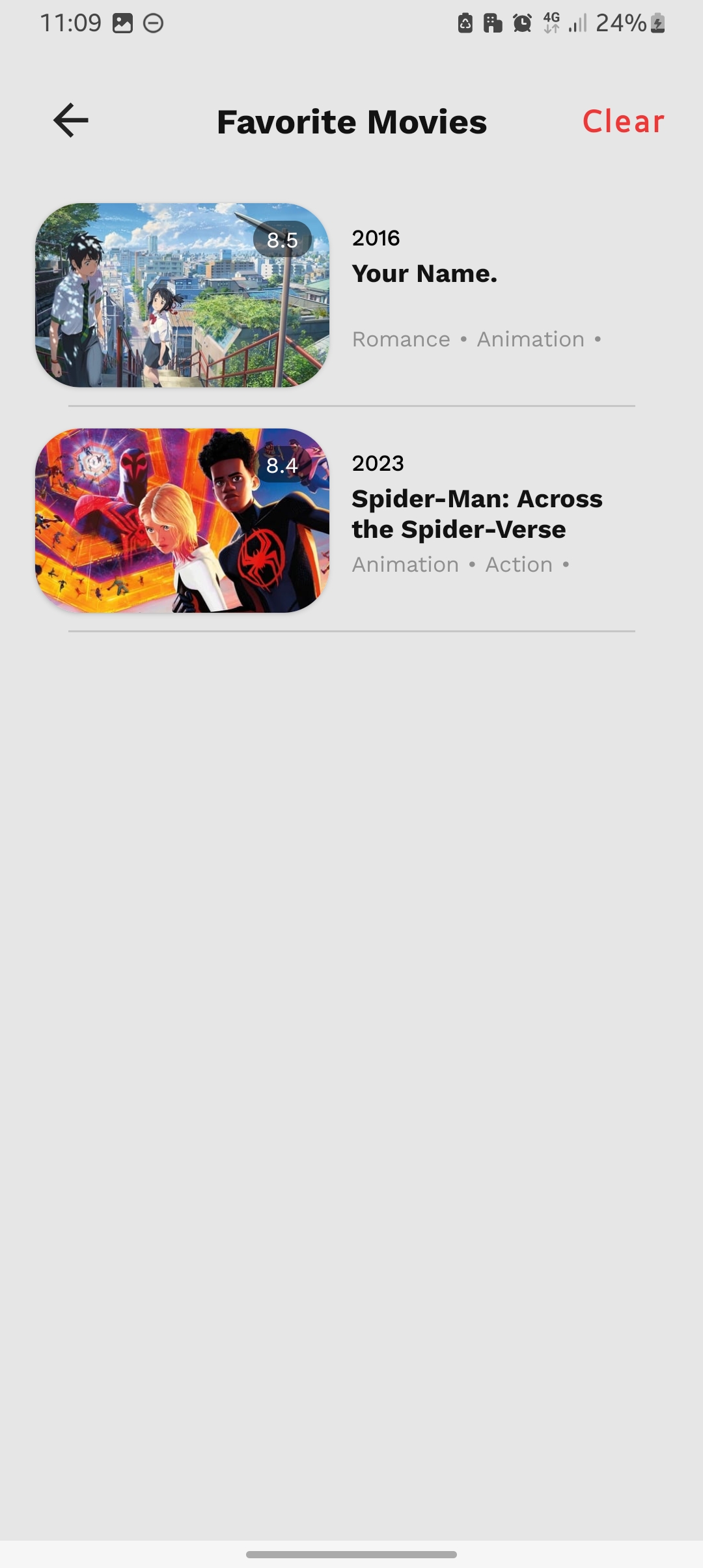
Provides in-depth information about the movie, including cast, release details, budget, trailers, and recommendations. You can also add movies to your favorites list from here
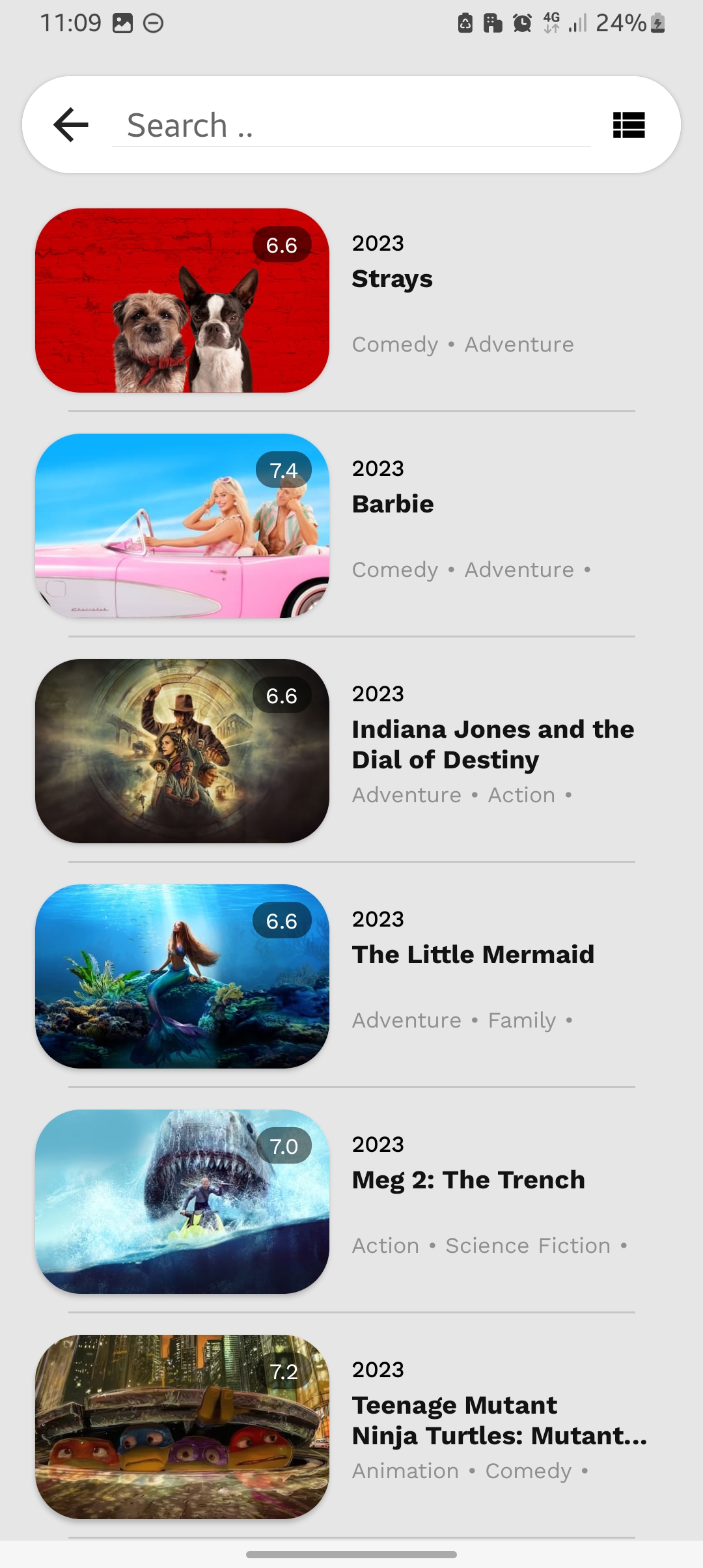
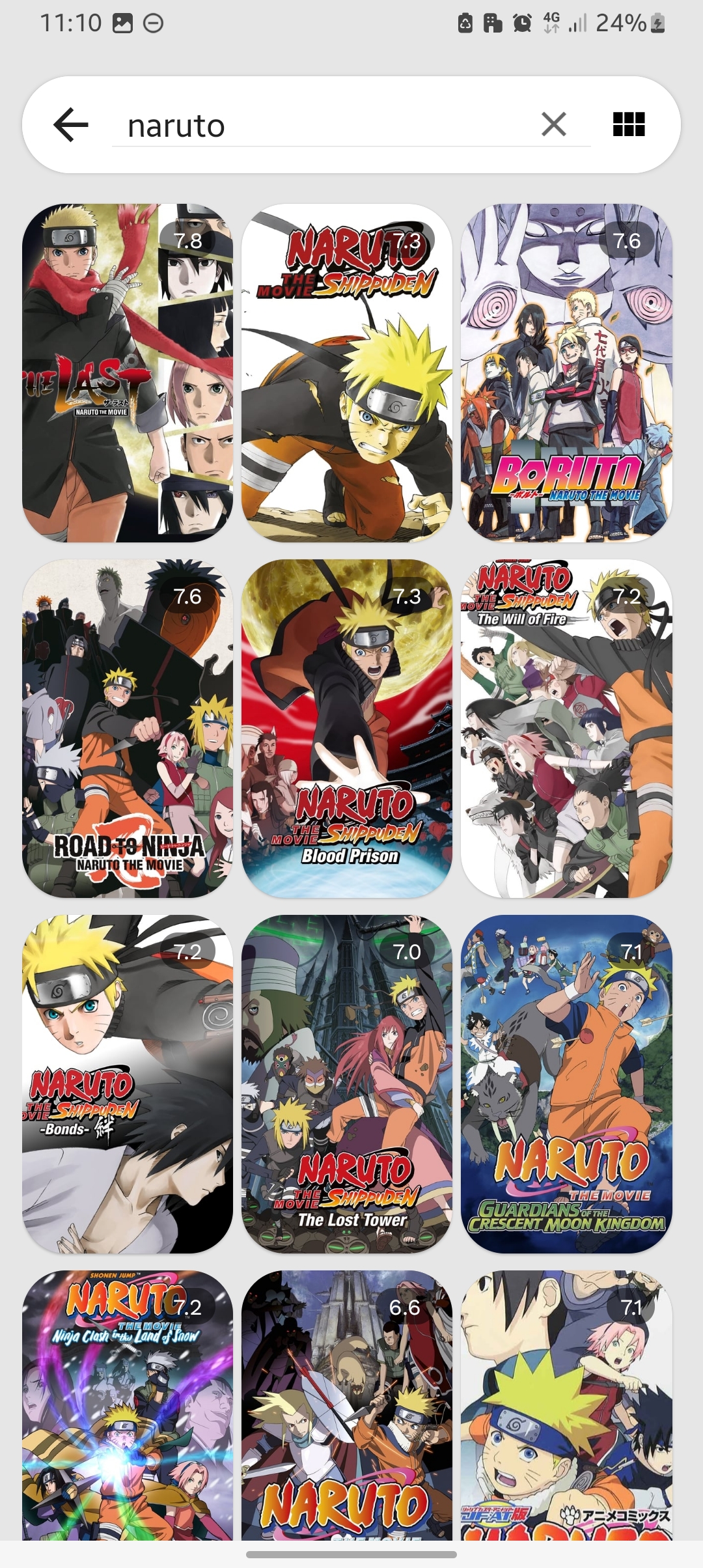
Utilize the search function to find movies that you like
Lesson Learned
I thoroughly enjoyed and learned a lot while developing this app. One thing I realized when trying to implement clean architecture is that it’s challenging to get it right in the first iteration. However, over time, as I added more features, it became much easier. This architecture ensures that each module and layer has its own responsibility and is loosely coupled from other modules. As a result, making changes to an existing feature or adding new ones is seamless, and the development velocity significantly improves.